The backbone of Salesforce: Apex part one Primitive Types
- caleksiev
- Aug 19, 2022
- 4 min read
What is Apex: it is a strongly typed, object-oriented programming language that allows developers to execute flow and transaction control statements on the Force.com platform, developed by Salesforce.
In order to write Apex code, you need two things:
- Sign up for free Salesforce Developer edition https://developer.salesforce.com/signup
- Once logged in your org ( short from organization ), go to Setup > Developer Console

That’s it, no additional IDE (integrated development environment) for now. Later on we will talk about VS Code for fast and free development and other tools like WebStorm and Illuminated Cloud 2, don’t worry about them for now.
In developer console go to Debug > Open Execute Anonymous Window
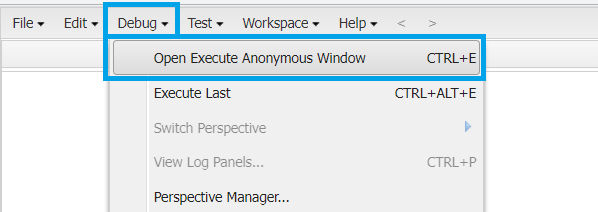
Here is our own universe for executing quick and small chunks of Apex code
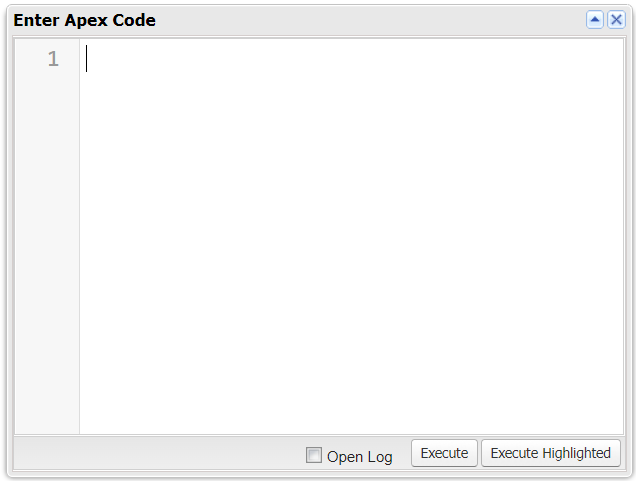
However, in order to start writing something, we first need to know Apex a little bit better. Let us dive in.
What does it mean that Apex is a strongly typed language? It means that every variable must be declared with the appropriate data type. Keep in mind that all Apex variables are initialized to null, so you should be assigning the proper value. If you do not assign a value to a variable and try to “call” it in your code, you will get an exception.
NOTE: We are not going to talk about the System class for now. Just know that, if we want to print something on the debug log ( where all the info about our code / transaction is ), we are going to use A LOT System.debug() method.
DATA TYPES:
- Primitive: Integer, Double, Long, Date, Datetime, String, ID, Boolean. There is Blob as well, but we are not going to talk about it for now
- sObject
- Collection: List ( array, in Apex it’s the same thing ), Set, Map
- Enum
- Null
Because there is quite a lot of information to cover, I will divide this in different posts, starting with the Primitive type. Also, please note that for most of the types there could be a stand - alone article ( like String data type for example ). I am going to show you just a simple example for each one of them and I will reave a reference after each data type to the official Salesforce Developer Guide
INTEGER:
32-bit number, does NOT include decimal point
MIN VALUE: - 2 147 483 648
MAX VALUE: 2 147 483 647
NOTE: there is a bug in Apex, which does not allow you to declare directly a variable to the min value, so keep in mind that, if you try that, you will get an error:
Integer min = -2147483648;
System.debug('INTEGER MIN VALUE: ' + min);
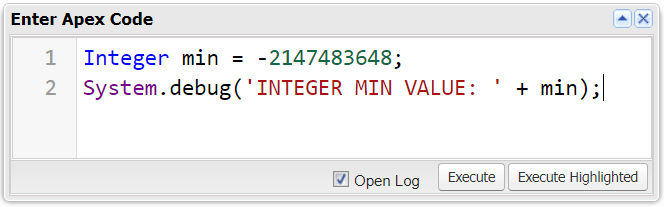
*Check the “Open Log” checkbox
*Click on “Execute” button in order to execute your code
Executing the above code will produce, because of the bug I told you about.
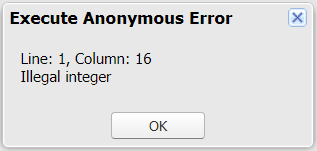
There is a workaround:
Integer min = Integer.valueOf('-2147483648');
System.debug('INTEGER MIN VALUE: ' + min);
Result:

Be sure to check the "Debug Only" checkbox at the bottom of your log, so that we can see only the USER_DEBUG ( or our System.debug() ) only:

We covered a lot so far and I know that some of the information is not needed at this point, but I believe that you should know about this bug.
DOUBLE:
64-bit number, includes decimal point
MIN VALUE: - (2^63)
MAX VALUE: 2^63-1
Double pi = 3.14159265359;
System.debug('Double value example: ' + pi);
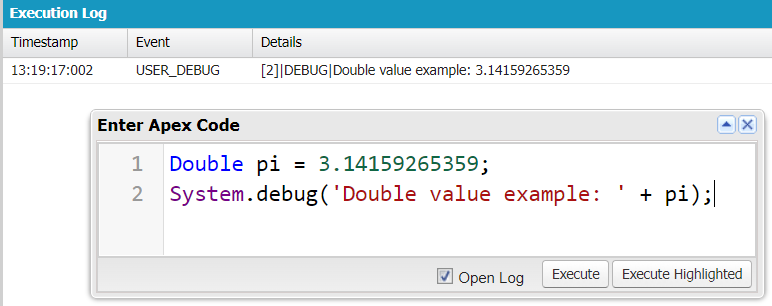
LONG:
64-bit number, does NOT include decimal point
MIN VALUE: - (2^63)
MAX VALUE: 2^63-1
NOTE: If the value of the number is less than or equal to Integer max / min value, in order to let Apex know that we really want to use a long variable, we should append 'L' letter after the number. Keep in mind that if you write a lower case 'l' will work as well, but in your code the number '1' and the letter 'l' can be mistaken very easily, so my advice is to always use capital 'L':
Long longValue = 314159261l;
System.debug('Long value example: ' + longValue);
Long longValueCapL = 314159261L;
System.debug('Long value example: ' + longValueCapL);
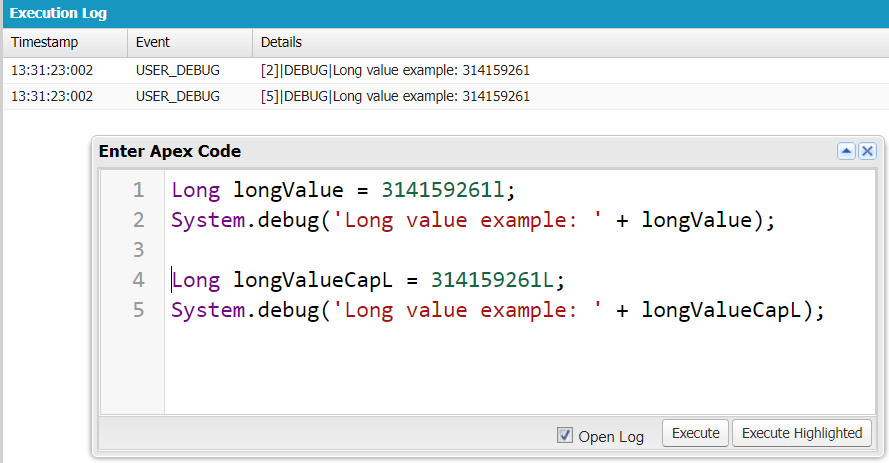
Reference: https://developer.salesforce.com/docs/atlas.en-us.apexref.meta/apexref/apex_methods_system_long.htm
DATE:
A value that indicates a particular day. We can add or subtract Integer values and get returned Date value. In order to create a date value we must use out of the box method, like this:
Date sampleDate = Date.newInstance(2022, 8, 15);
System.debug('My sample date: ' + sampleDate);
//we will try to add 5 days to our date
Date myNewDate = sampleDate.addDays(5);
System.debug('My sample date with 5 days added: ' + myNewDate);
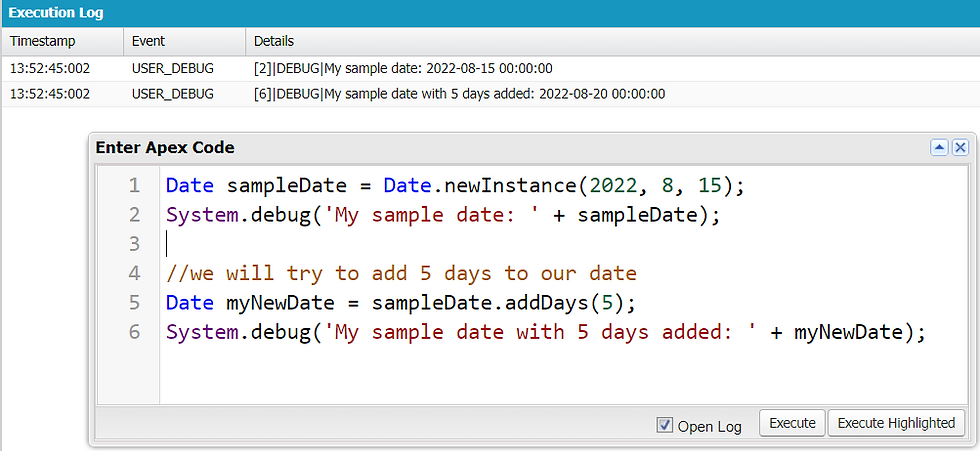
DATETIME:
A value that indicates a particular day and time. We can add or subtract an Integer or Double value from a Datetime value, returning a Date value. In order to create a datetime value we must use out of the box method, like this:
//Parameters: Integer year, Integer month, Integer day, Integer hour, Integer minute, Integer second
Datetime sampleDateTime = Datetime.newInstanceGmt(2022, 8, 15, 11, 25, 15);
System.debug('My sample DATE TIME: ' + sampleDateTime);
Datetime dateTimeAddedHours = sampleDateTime.addHours(6);
System.debug('My DATE TIME with added hours: ' + dateTimeAddedHours);

STRING:
A set of characters, surrounded by single quotes.
NOTE: there are really a lot of system methods for you to choose from for any situation you can think of.
String myTestString = 'This is my string and it could be very long, depending on the Salesforce heap size limit';
System.debug('MY TEST STRING: ' + myTestString);
System.debug('IF IT CONTAINS \'Salesforce\': ' + myTestString.containsIgnoreCase('salesforce'));
System.debug('All white spaces deleted: ' + myTestString.deleteWhitespace());
System.debug('Does it ends it \'LIMIT\': ' + myTestString.endsWithIgnoreCase('limit'));
System.debug('Everything to upper case: ' + myTestString.toUpperCase());
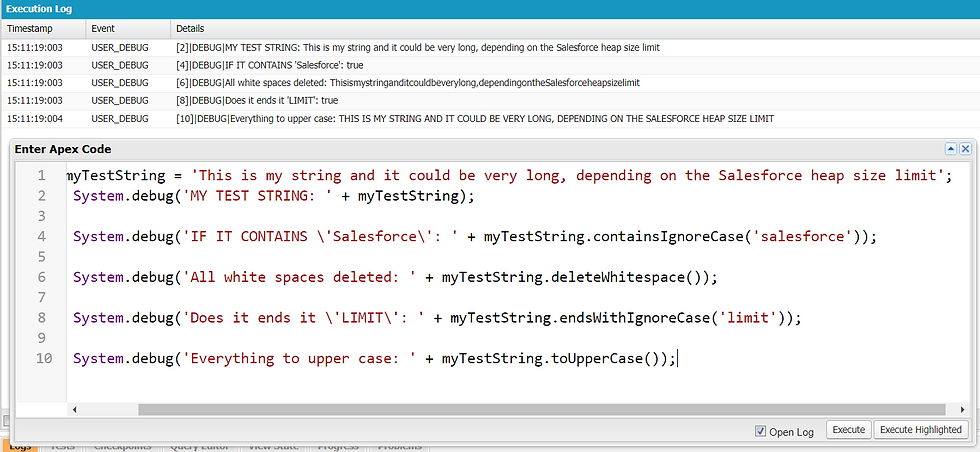
ID:
A valid unique 18-character Lightning Platform record identifier. If the ID is set to a 15-character value, Apex converts the value to its 18-character representation. All invalid ID values are rejected with a runtime exception.
Switch to your Salesforce org tab, click on any tab, for example "Accounts", than from the drop down select "All Accounts" and click on the first account record in your table ( it might be different in your org ):
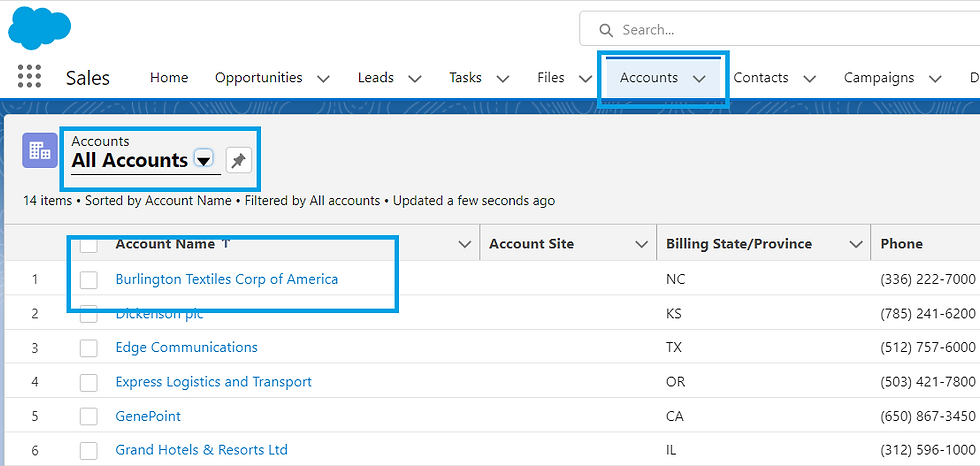
Now, you are in the clicked account details page and in the URL you can see the ID of the record you are in:
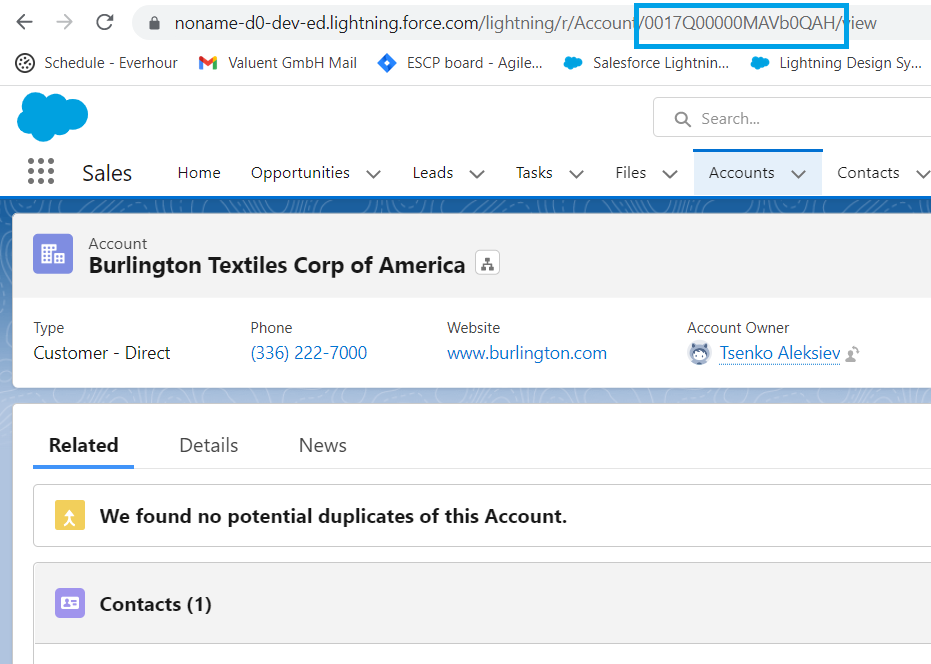
Id accountId = '0017Q00000MAVb0QAH';
System.debug('My account ID: ' + accountId);
System.debug('MY ID sObject Type: ' + accountId.getSobjectType());
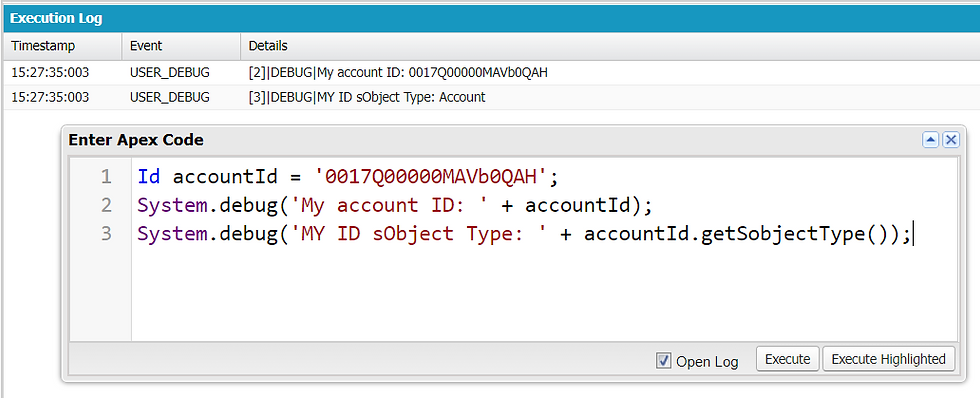
Reference: https://developer.salesforce.com/docs/atlas.en-us.apexref.meta/apexref/apex_methods_system_id.htm
BOOLEAN:
Values that can be assigned:
TRUE
FALSE
NULL
Usually this data type is used to set a flag if a condition is met or not
Boolean doYouLike = true;
System.debug('Do you like Salesforce: ' + doYouLike);
doYouLike = false;
System.debug('Do you like learning alone: ' + doYouLike);
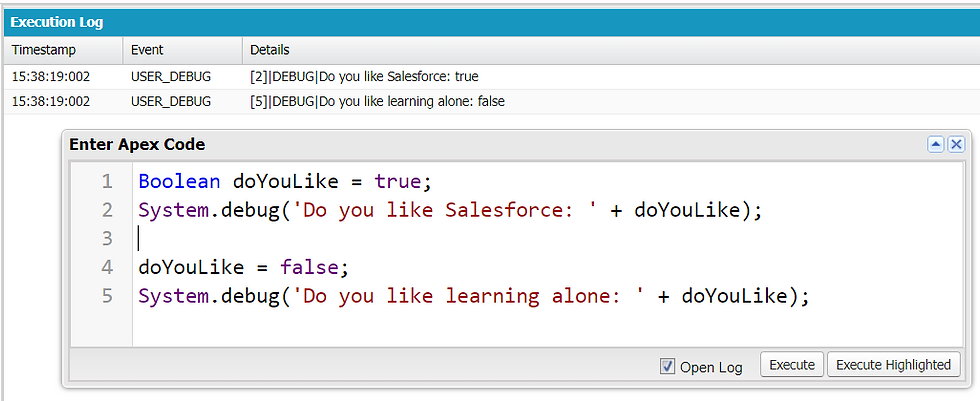
to be continued: Apex Part Two sObject
Comments